Being NetBeans my favourite IDE, as you might have suspected, I'll try to jot down some notes on how to use it to create a very simple Naked Objects application.
First things first, you have to download the Naked Objects framework; you have various options, I chose to download the zip file for Ant. After unzipping the file you should find a folder named lib that contains - o great wonder and surprise - all the necessary libs.
As I suppose I could use all this stuff in more than one project, I created a new library in NetBeans:
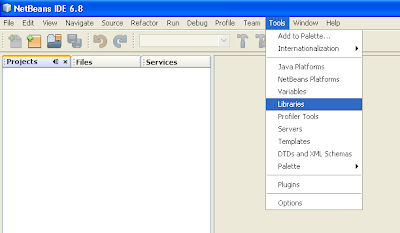
Click on the New Library... button and you'll be prompted a library name and type
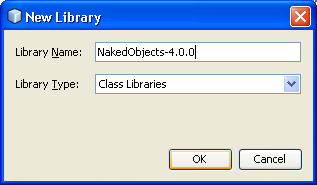
Then click on the Add Jar/Folder... button and add all the jars in the lib folder. You should get something like this:
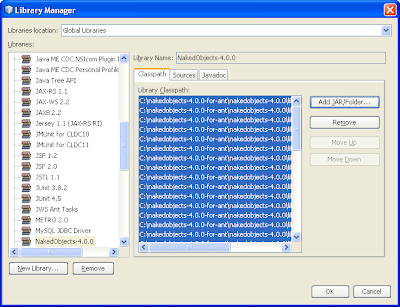
Ok, now we can proceed to creating a new application. As a disclaimer, this is not necessarily the best way to do it, but it is how my inner cogs work (or don't work). If yours are different, you'll have to compose all the pieces of the puzzle in a different order.
Let's suppose we want to use the DnD GUI, so I go with a new Java Application:
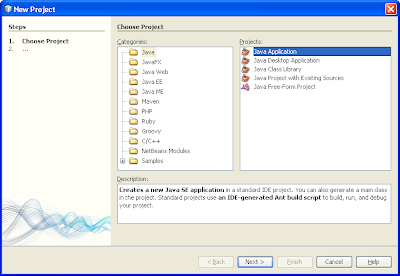
Click on the Next> button and enter a name for your project; don't use a dedicated folder for libraries and don't have the IDE create a main class for you.
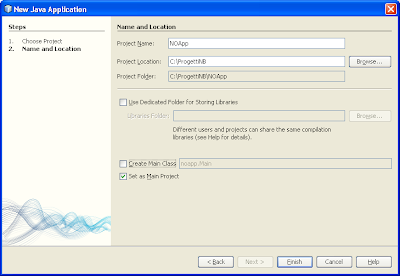
Click on the Finish button. Ok, now we should instruct our project to be a Naked Object Application. Right click the Libraries node to add all necessary references:
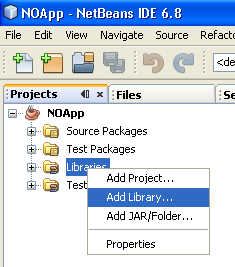
Select the library you've previosly created and you're almost ready to go.
Now, if you try to run the application NetBeans complains because you have not chosen a Main Class. Right click the project node and the Run node in the Categories tree, and insert org.nakedobjects.runtime.NakedObjects as the Main Class.
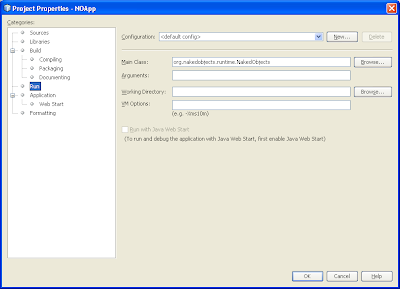
Click on the OK button. Ok, now we can try to run the application... just to get another complain
Exception in thread "main" org.nakedobjects.metamodel.commons.exceptions.NakedObjectException: failed to load 'nakedobjects.properties'; tried using: [file system (directory 'config'), file system (directory 'src/main/webapp/WEB-INF'), context loader classpath]
It seems fair... after all we have to provide some configuration. Create a new properties file, name it nakedobjects and put it in the config folder. Obviously this is not enough, and you can check it yourself trying to run the application: NetBeans will complain again (don't shoot the messenger) about the lack of services.
To define services, we have to write some code. Let's suppose we want to deal with all Walt Disney characters - I hope there are no legal issues with this - so let's create a class that represents one:
package it.moz.noapp;
import org.nakedobjects.applib.AbstractDomainObject;
import org.nakedobjects.applib.annotation.DescribedAs;
import org.nakedobjects.applib.annotation.MemberOrder;
@DescribedAs("Character")
public class Character extends AbstractDomainObject {
private String firstname;
private String lastName;
@MemberOrder(sequence = "1")
public String getFirstname() {
resolve(firstname);
return firstname;
}
public void setFirstname(String firstname) {
this.firstname = firstname;
objectChanged();
}
@MemberOrder(sequence = "2")
public String getLastName() {
resolve(lastName);
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
objectChanged();
}
public String title() {
return getFirstname() + " " + getLastName();
}
}
Notice how this class, that is a domain object, extends the class AbstractDomainObject provided by the framework. The annotation @DescribedAs tells you how the object will be referred to in the GUI, while @MemberOrder defines the order in which members are presented. You can find every detail on the Naked Objects website.
The next step is telling our application that such a domain object exists, so we'll add the following line in our nakedobject.properties file:
nakedobjects.services=repository#it.moz.noapp.Character
Ok, let's start the application...
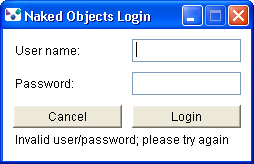
uh oh... User name and password? ehrm... let's provide the application with them! The problem is that if you want to add an empty file - unlike for properties files - NetBeans asks you whether you want to add it to the src folder or to the test folder, i.e. the folders (already) defined in the project. As you want neither, you must manually add an empty passwords file to the config folder. Before complaining about NetBeans, keep in mind that you can always define the config folder as a new source package folder, but let's skip this option.
Fill in a username and a password...
user:passAnd that's it, you can start your application!
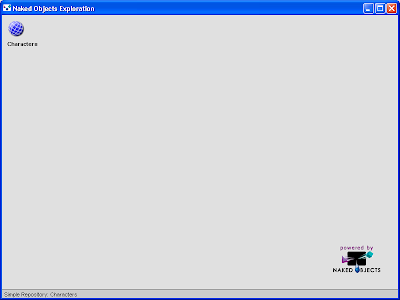
To create a new instance you right-click the Characters icon and select the appropriate item from the drop down list, insert the desired values and here's your brand new character. Rinse and repeat and you'll get something like this:
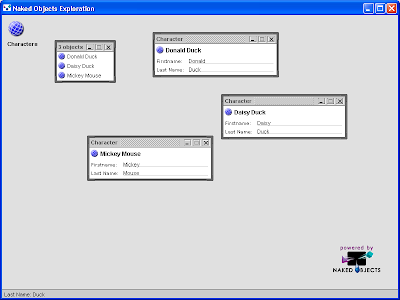
As you can see, you now have a Disney Character directory without having written a single line of code but your domain class.
Unluckily, if you close your application all your efforts have been vain, so you should add the support for persistence. But this will be the subject for another post...